API Keys are used to authenticate all requests against Conekta's api using the Bearer Authentication. API Keys can be recovered from Conekta's Panel. Each key is configured for either sandbox or production modes, and each mode will have a public key for frontend card tokenization and private key for making secure backend requests..
Never share your private keys with anyone as it will allow them complete access to your account.
Sandbox mode allows you to simulate payment flows without actually completing payments. You can find more information about possible test cards and scenarios in the Cards Test Kit Throughout our documentation we have provided examples with sandbox keys so that you can test your integration without creating a Conekta account.
For practicality we suggest using out official libraries, which are regularly updated to simply integration with the most recent version of the API in the following programming languages:
Remember that:
-
You can obtain you private key in Conekta's Panel
- Key_LlavePRIVADA123456789
-
You can use the following header to authenticate yourself
- Authorization: Bearer Key_LlavePRIVADA123456789
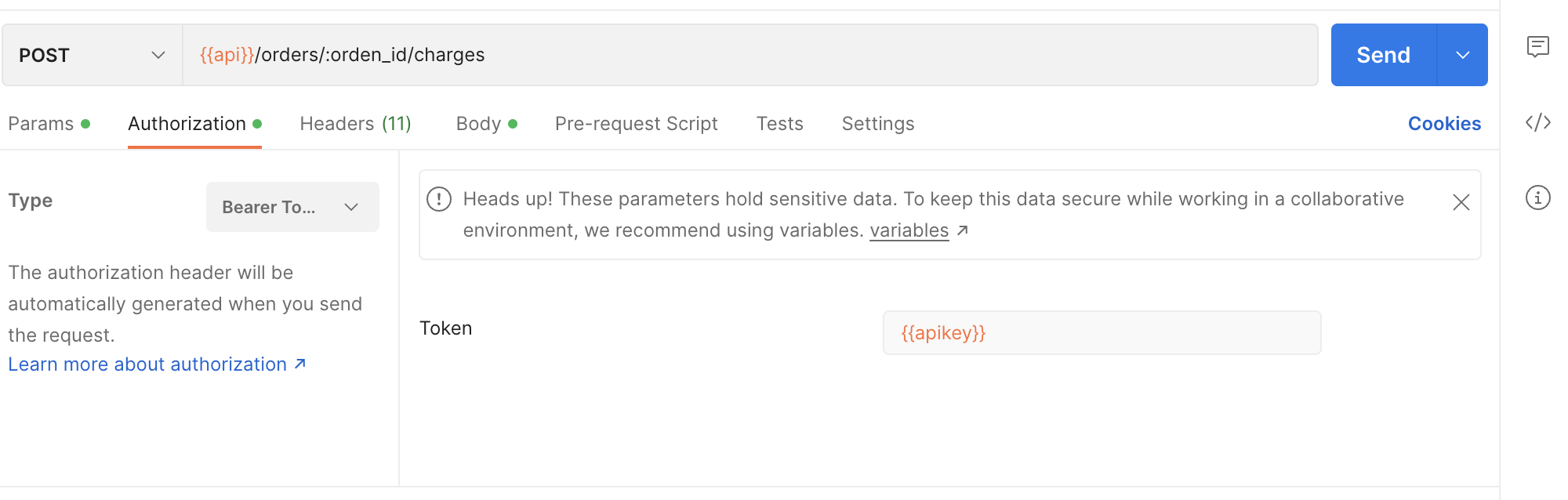
Image 1: An example of how the authentication key can be set in Postman's Authorization section using an environment variable
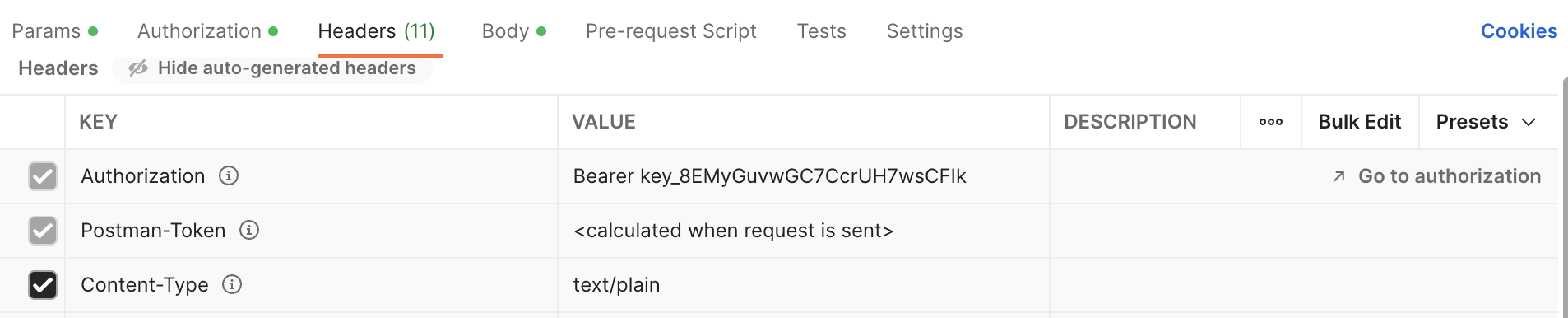
Image 2: An example of how the Postman authentication key will be rendered as a header
curl --location --request POST 'https://api.conekta.io'/{API_endpoint}' \
//POST / PUT / GET / DEL son admitidas dependiendo del endpont a utilizar//
--header 'Accept: application/vnd.conekta-v2.1.0+json' \
--header 'Accept-Language: es' \
--header 'Content-Type: application/json' \
-u key_xxxxxxxxxxxxxx: \
--data-raw '{
//JSON REQUEST BODY
}'
require "uri"
require "json"
require "net/http"
url = URI("https://api.conekta.io/orders/{{orden_id}}/charges")
https = Net::HTTP.new(url.host, url.port)
https.use_ssl = true
request = Net::HTTP::Post.new(url)
request["Accept"] = "application/vnd.conekta-v2.1.0+json"
request["Content-type"] = "application/json"
request["Authorization"] = "Bearer key_xxxxxxx"
request.body = JSON.dump({})
response = https.request(request)
puts response.read_body
<?php
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://api.conekta.io/charges',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'POST',
CURLOPT_HTTPHEADER => array(
'Accept: application/vnd.conekta-v2.1.0+json',
'Content-type: application/json',
'Authorization: Bearer key_xxxxxx'
),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
import requests
import json
url = "https://api.conekta.io/orders/{{orden_id}}/charges"
payload = ""
headers = {
'Accept': 'application/vnd.conekta-v2.1.0+json',
'Content-type': 'application/json',
'Authorization': 'Bearer key_xxxxx'
}
response = requests.request("POST", url, headers=headers, data=payload)
print(response.text)
var axios = require('axios');
var data = '';
var config = {
method: 'post',
maxBodyLength: Infinity,
url: 'https://api.conekta.io/orders/{{orden_id}}/charges',
headers: {
'Accept': 'application/vnd.conekta-v2.1.0+json',
'Content-type': 'application/json',
'Authorization': 'Bearer key_xxxxx'
},
data : data
};
axios(config)
.then(function (response) {
console.log(JSON.stringify(response.data));
})
.catch(function (error) {
console.log(error);
});
OkHttpClient client = new OkHttpClient().newBuilder()
.build();
MediaType mediaType = MediaType.parse("application/json");
RequestBody body = RequestBody.create(mediaType, "");
Request request = new Request.Builder()
.url("https://api.conekta.io/orders/{{orden_id}}/charges")
.method("POST", body)
.addHeader("Accept", "application/vnd.conekta-v2.1.0+json")
.addHeader("Content-type", "application/json")
.addHeader("Authorization", "Bearer key_xxxxxx")
.build();
Response response = client.newCall(request).execute();
var client = new HttpClient();
var request = new HttpRequestMessage(HttpMethod.Post, "https://api.conekta.io/orders/{{orden_id}}/charges");
request.Headers.Add("Accept", "application/vnd.conekta-v2.1.0+json");
request.Headers.Add("Content-type", "application/json");
request.Headers.Add("Authorization", "Bearer key_xxxxx");
var content = new StringContent("", null, "application/json");
request.Content = content;
var response = await client.SendAsync(request);
response.EnsureSuccessStatusCode();
Console.WriteLine(await response.Content.ReadAsStringAsync());
package main
import (
"fmt"
"strings"
"net/http"
"io/ioutil"
)
func main() {
url := "https://api.conekta.io/orders/%7B%7Borden_id%7D%7D/charges"
method := "POST"
payload := strings.NewReader(``)
client := &http.Client {
}
req, err := http.NewRequest(method, url, payload)
if err != nil {
fmt.Println(err)
return
}
req.Header.Add("Accept", "application/vnd.conekta-v2.1.0+json")
req.Header.Add("Content-type", "application/json")
req.Header.Add("Authorization", "Bearer key_xxxxx")
res, err := client.Do(req)
if err != nil {
fmt.Println(err)
return
}
defer res.Body.Close()
body, err := ioutil.ReadAll(res.Body)
if err != nil {
fmt.Println(err)
return
}
fmt.Println(string(body))
}